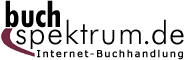 Neuerscheinungen 2016Stand: 2020-02-01 |
Schnellsuche
ISBN/Stichwort/Autor
|
Herderstraße 10 10625 Berlin Tel.: 030 315 714 16 Fax 030 315 714 14 info@buchspektrum.de |
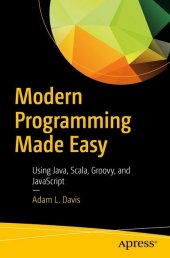
Adam L. Davis
Modern Programming Made Easy
Using Java, Scala, Groovy, and JavaScript
1st ed. 2016. xvii, 126 S. 52 SW-Abb., 8 Farbabb. 235 mm
Verlag/Jahr: SPRINGER, BERLIN; APRESS 2016
ISBN: 1-484-22489-2 (1484224892)
Neue ISBN: 978-1-484-22489-2 (9781484224892)
Preis und Lieferzeit: Bitte klicken
Get up and running fast with the basics of programming using Java as an example language. This short book gets you thinking like a programmer in an easy and entertaining way. Modern Programming Made Easy teaches you basic coding principles, including working with lists, sets, arrays, and maps; coding in the object-oriented style; and writing a web application.
This book is language agnostic, but will mainly cover Java, with some references to Groovy, Scala, and JavaScript to give you a broad range of examples to consider. You will get a taste of what modern programming has to offer and set yourself up for further study and growth in your chosen language.
What You´ll Learn
Code using the functional programming style
Build and test your code
Read and write from files
Design user interfaces
Deploy your app in the cloud
Who This Book Is For<
Anyone who wants to learn how to code. Whether you´re a student, a teacher, looking for a career change, or just a hobbyist, this book is made for you.
Starting Out 1. Introduction 1.1 Problem Solving 1.2 This Book 2. Software to Install 2.1 Java/Groovy 2.2 Others 2.3 Code on Github 3. The Basics 3.1 Coding Terms 3.2 Primitives and Reference 3.3 Strings/Declarations 3.4 Statements 3.5 Assignment 3.6 Class and Object 3.7 Comments 3.8 Summary Glorified Calculator 4. Math 4.1 Adding, subtracting, etc.< 4.2 More complex Math 4.3 Random numbers 4.4 Summary 5. Arrays, Lists, Sets, and Maps 5.1 Arrays 5.2 Lists 5.3 Sets 5.4 Maps 5.5 Summary 6. Conditionals and Loops< 6.1 If, Then, Else 6.2 Switch Statements 6.3 Boolean logic 6.4 Looping 6.5 Summary 7. Methods 7.1 Call me 7.2 Break it down 7.3 Return to sender 7.4 Static 7.5 Varargs 7.6 Main method 7.7 Exercises 7.8 Summary Polymorphic Spree 8. Inheritance 8.1 Objectify 8.2 Parenting 101 8.3 Packages 8.4 Public Parts 8.5 Interfaces 8.6 Abstract Class 8.7 Enums 8.8 Annotations 8.9 Autoboxing 8.10 Summary 9. Design Patterns 9.1 Observer 9.2 MVC 9.3 DSL 9.4 Actors 10. Functional Programming 10.1 Functions and Closures 10.2 Map/Filter/etc. 10.3 Immutability 10.4 Java 8 10.5 Groovy 10.6 Scala 10.7 Summary 11. Refactoring 11.1 Object-Oriented Refactoring 11.2 Functional Refactoring 11.3 Refactoring Examples 12. Utilities 12.1 Dates and Times 12.2 Currency 12.3 TimeZone 12.4 Scanner Real-life 13. Building 13.1 Ant 13.2 Maven 13.3 Gradle 14. Testing 14.1 Types of Tests 14.2 JUnit 15. Input/Output 15.1 Files 15.2 Reading Files 15.3 Writing Files 15.4 Downloading Files 15.5 Summary 16. Version Control 16.1 Subversion 16.2 Git 16.3 Mercurial 17. The Inter-webs 17.1 Web 101 17.2 My First Web-app 17.3 The Holy Grails 17.4 Cloud 17.5 The REST 17.6 Summary 18. Swinging Graphics 18.1 Hello Window 18.2 Push my Buttons 18.3 Fake Browser 18.4 Griffon 18.5 Advanced Graphics 18.6 Graphics Glossary 18.7 Summary 19. Creating a Magical User Experience 19.1 Application Hierarchy19.2 Consider your Audience 19.3 Choice is an Illusion 19.4 Direction 19.5 Skuemorphism 19.6 Context is Important 19.7 KISS 19.8 You Are Not the User 19.9 Summary 20. Databases 20.1 SQL (Relational) Databases 20.2 NoSQL Databases 20.3 Summary 21. Conclusion Appendixes Java/Groovy Java/Scala Java/JavaScript Resources Free Online Learning The Death of College? Money Betting on the Student More Online Resources Java